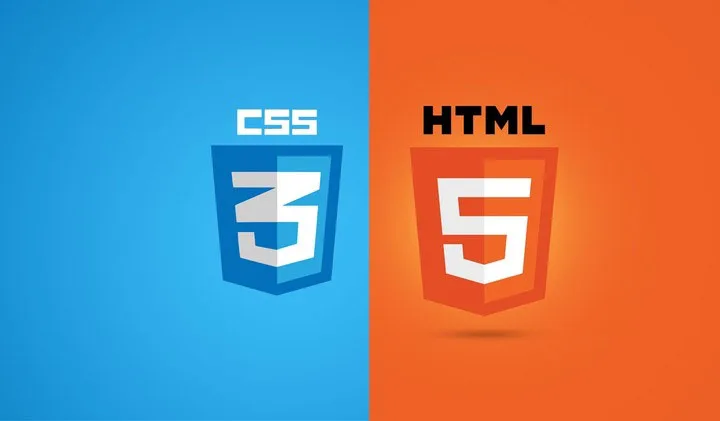
As we dive deeper into the world of web development, we often get caught up in the more complex concepts—JavaScript, frameworks, APIs, and all that jazz. But sometimes, the simplest tools and tricks in HTML and CSS can make your development life so much easier.
If you’re looking to level up your front-end skills, here are 10 HTML & CSS tricks that every developer should know. These aren’t just cool to know—they’re game-changers that’ll help you work faster, build more responsive sites, and create better designs.
Let’s dive in!
1. The Magic of Flexbox for Layouts
Flexbox is the layout tool we all needed but didn’t know we wanted. It lets you design flexible, responsive layouts with minimal code.
If you’ve ever struggled with centering items or arranging elements in a row or column, flexbox has your back. For example:
.container { display: flex; justify-content: center; /* Centers content horizontally */ align-items: center; /* Centers content vertically */ }
This simple code will center everything inside .container
—both horizontally and vertically—without breaking a sweat.
2. CSS Grid for Complex Layouts
While flexbox handles one-dimensional layouts (row or column), CSS Grid is your best friend when you need two-dimensional control over your layout.
Want a clean, structured layout with rows and columns? With CSS Grid, it’s as easy as pie:
.container { display: grid; grid-template-columns: repeat(3, 1fr); /* 3 equal-width columns */ grid-gap: 10px; }
With just a few lines of CSS, you can create a neat 3-column grid. No more mess with floats or weird calculations!
3. Centering Content Made Simple
Centering elements is one of the trickiest problems to solve, but with flexbox, it’s easy as pie.
Want to center an element both vertically and horizontally? Just use:
.container { display: flex; justify-content: center; align-items: center; height: 100vh; /* Full viewport height */ }
That’s it! You can now center anything—buttons, text, images—effortlessly, regardless of the screen size.
4. Style Specific Elements with :nth-child
Sometimes, you want to target a specific child element in a list or container without adding extra classes. Enter the :nth-child
pseudo-class.
Want to style every odd-numbered list item differently? Here’s how:
ul li:nth-child(odd) { background-color: #f0f0f0; }
With this selector, you can style items based on their position, making it a super flexible way to target elements dynamically.
5. Customizing Form Elements
Let’s be honest—default form elements are pretty bland. But with a little CSS magic, you can create customized buttons, checkboxes, and inputs that match your site’s design.
For instance, you can change the color of a checkbox like this:
input[type="checkbox"] { width: 20px; height: 20px; accent-color: blue; }
This gives you more control over how your form elements look, helping your forms blend seamlessly into your design.
6. Responsive Web Design with Media Queries
We live in a mobile-first world, which means your site needs to look great on every screen. Media queries allow you to adapt your layout and design based on the screen size.
For example, if you want to switch your layout to a column on smaller screens, use:
@media (max-width: 768px) { .container { flex-direction: column; /* Stack items vertically on small screens */ } }
Media queries make it easy to create responsive designs that adjust to any screen size—from mobile phones to desktops.
7. Positioning Made Easy with position
CSS positioning is one of those concepts that can be tricky at first, but once you understand it, it opens up a world of possibilities.
The position
property allows you to place elements exactly where you want them:
.container { position: relative; } .box { position: absolute; top: 10px; left: 10px; }
This code will position the .box
element 10px from the top-left corner of its nearest positioned ancestor. You can get really precise with positioning when you need to!
8. Accessible Websites with ARIA
Making websites accessible is crucial. One way to enhance accessibility is by using ARIA (Accessible Rich Internet Applications) attributes. These help screen readers understand your content better, making your website more inclusive.
For example, if you have a button that expands a menu, you can use aria-expanded
to indicate whether the menu is open:
<button aria-expanded="false">Open Menu</button>
ARIA makes it easier to make your site accessible to all users, including those with disabilities.
9. CSS Transitions and Animations
Want to add some life to your site? CSS transitions and animations can make your website feel more interactive and polished.
For a simple hover effect:
button { transition: background-color 0.3s ease; } button:hover { background-color: #3498db; /* Smooth color change */ }
With just a few lines of CSS, you can make your site feel way more dynamic and engaging.
10. Using CSS Variables (Custom Properties)
CSS Variables, or custom properties, allow you to store values like colors, fonts, and spacing to use throughout your stylesheet. This helps make your code more maintainable.
For example:
:root { --main-color: #3498db; } .button { background-color: var(--main-color); }
By defining your values in one place (like :root
), you can easily tweak them later without hunting through your entire CSS file.
Conclusion
And there you have it—10 simple but powerful HTML & CSS tricks that every developer should know. These tricks will not only make your coding life easier but also help you create more responsive, beautiful websites that work across devices and browsers.
Remember, coding is all about learning and improving. So, the next time you sit down to code, try incorporating some of these tricks and see how much easier and fun it becomes!
Stay tuned for more tips and tutorials here on CodingNaija! Happy coding!