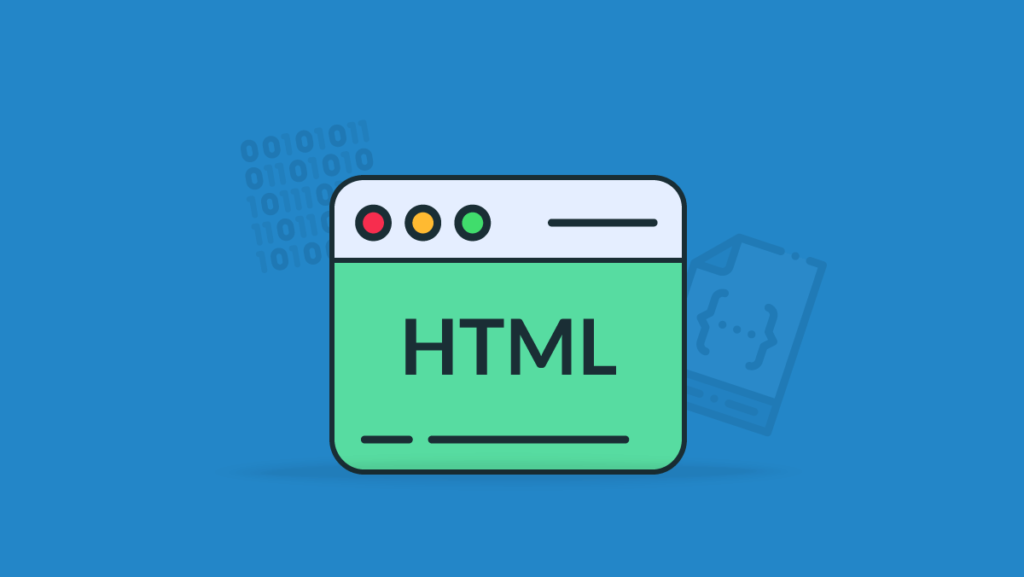
HTML and CSS are the backbone of web development. As simple as they may seem at first glance, they come with their own set of challenges that can trip up even experienced developers. In this post, we’ll explore the most common mistakes developers make when working with HTML and CSS, and I’ll share practical tips to help you avoid them. Whether you’re a newbie or looking to refine your skills, this guide is for you.
Common Pitfalls in HTML and CSS
1. Ignoring Semantic HTML
Using <div>
and <span>
for everything may seem convenient, but it’s a missed opportunity to make your code more meaningful and accessible.
Example:
<!-- Wrong --> <div>Header</div> <div>Article Content</div> <div>Footer</div> <!-- Correct --> <header>Header</header> <article>Article Content</article> <footer>Footer</footer>
Tip: Use tags like <header>
, <section>
, and <footer>
to structure your content properly.
2. Forgetting to Close Tags
Leaving tags unclosed can wreak havoc on your layout.
Example:
<!-- Wrong --> <p>This is a paragraph <!-- Correct --> <p>This is a paragraph</p>
Tip: Always double-check that your opening tags have corresponding closing tags.
3. Misunderstanding the Box Model
The box model can be confusing, especially when margins, paddings, and borders affect your element’s size in unexpected ways.
Example:
<style> div { width: 100px; padding: 10px; border: 5px solid black; box-sizing: border-box; /* Ensures total width remains 100px */ } </style> <div>Box Model Example</div>
Tip: Use box-sizing: border-box;
to make width and height calculations predictable.
4. Overcomplicating Z-Index
Randomly assigning high z-index
values without understanding stacking contexts can lead to chaos.
Example:
<style> .box1 { position: absolute; z-index: 10; background-color: red; width: 100px; height: 100px; } .box2 { position: absolute; z-index: 5; background-color: blue; width: 100px; height: 100px; top: 20px; left: 20px; } </style> <div class="box1"></div> <div class="box2"></div>
Here, .box1
will appear on top because of its higher z-index
.
Tip: Plan your z-index hierarchy carefully.
5. Neglecting Responsive Design
Hardcoding pixel values without using media queries can make your site look broken on smaller screens.
Example:
<style> .container { width: 100%; } @media (min-width: 600px) { .container { width: 80%; /* Adjusts for larger screens */ } } </style> <div class="container">Responsive Box</div>
Tip: Use relative units (%
, em
, rem
) and media queries to make your design flexible.
6. Skipping Alt Attributes on Images
Accessibility and SEO take a hit when alt
attributes are missing.
Example:
<img src="image.jpg" alt="A beautiful sunset over the mountains">
Tip: Always include descriptive alt
text for images.
7. Overusing Inline Styles
Inline styles make your code messy and hard to maintain.
Example:
<!-- Wrong --> <div style="color: red; font-size: 20px;">Inline Styles</div> <!-- Correct --> <style> .styled-text { color: red; font-size: 20px; } </style> <div class="styled-text">External Styles</div>
Tip: Keep styles in a separate stylesheet or <style>
block.
8. Overlooking Accessibility
Ignoring keyboard navigation and color contrast can exclude many users.
Example:
<button>Click Me</button> <a href="#section1">Go to Section 1</a> <input type="text" placeholder="Enter your name">
Tip: Ensure all interactive elements are keyboard-friendly and use tools like WebAIM Contrast Checker.
9. Ignoring Cross-Browser Compatibility
Modern features like grid
aren’t always supported in older browsers.
Example:
<style> .container { display: flex; /* Fallback for older browsers */ display: grid; /* Preferred for modern browsers */ grid-template-columns: 1fr 1fr; } </style> <div class="container"> <div>Item 1</div> <div>Item 2</div> </div>
Tip: Use Can I Use to check browser compatibility and provide fallbacks when necessary.
Expert Tips to Avoid These Pitfalls
- Plan Your HTML Structure: Use semantic tags to improve readability and accessibility.
- Validate Your Code: Tools like W3C Validator catch syntax errors.
- Keep Code Organized: Use consistent indentation and meaningful class names.
- Test on Multiple Devices: Use browser developer tools to simulate various screen sizes.
- Stick to External Stylesheets: Avoid inline styles for cleaner, reusable code.
- Learn the Box Model: Practice with
box-sizing
to master layout control. - Write Responsive Code: Think mobile-first and scale up with media queries.
- Check Accessibility Early: Use ARIA roles, keyboard navigation, and proper contrast ratios.
By being mindful of these common pitfalls and applying the tips shared here, you’ll write cleaner, more professional HTML and CSS. Whether you’re working on a personal project or the next big website for your client, following these best practices will set you apart as a skilled developer.