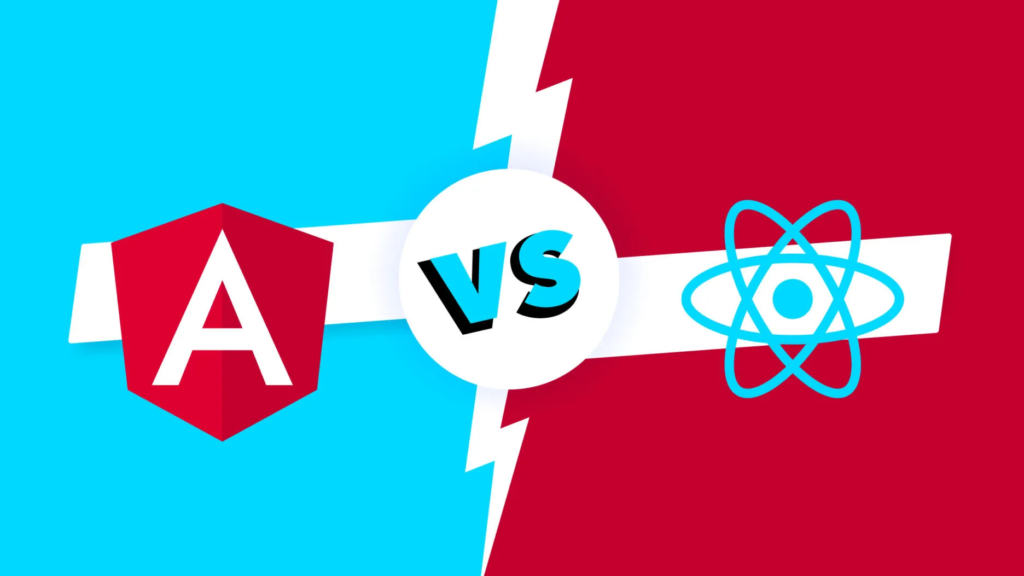
When it comes to frontend development, choosing the right framework is a big deal. It’s like picking the perfect tool for a job—your choice can make or break your project’s efficiency, scalability, and performance. Among the crowd of JavaScript frameworks, React and Angular consistently rise to the top. But which one should you go for? Let’s break it down and explore these two titans in detail.
React
React is a JavaScript library developed by Meta (formerly Facebook). It’s famous for its flexibility and its focus on building dynamic and interactive user interfaces. Unlike full-fledged frameworks, React gives you the building blocks for your application, letting you decide how to assemble them.
- Use Case: Dynamic web apps, mobile apps (React Native), and even VR (React 360).
- Core Features: JSX (JavaScript XML), components, and hooks for state management.
- Ecosystem: React works seamlessly with libraries like Redux, Next.js, and Material-UI, offering endless possibilities.
Angular
Angular, on the other hand, is a full-fledged framework developed by Google. It’s an opinionated tool that comes with everything you need to build large-scale applications, right out of the box. If React is a flexible Lego set, Angular is a pre-assembled powerhouse.
- Use Case: Enterprise-level apps, single-page applications (SPAs), and complex web apps.
- Core Features: TypeScript integration, dependency injection, and RxJS for reactive programming.
- Ecosystem: Angular’s built-in CLI and tools like Angular Universal make it highly productive.
File Structure and Code Examples
React File Structure
React projects typically have a minimal file structure, giving developers the freedom to organize files as they see fit. A basic structure might look like this:
my-react-app/ ├── src/ │ ├── components/ │ │ └── Header.js │ ├── App.js │ ├── index.js ├── public/ ├── package.json
React Code Example:
import React, { useState } from 'react'; function App() { const [count, setCount] = useState(0); return ( <div> <h1>Count: {count}</h1> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); } export default App;
Angular File Structure
Angular projects have a more defined structure, which is great for large teams. A typical Angular app looks like this:
my-angular-app/ ├── src/ │ ├── app/ │ │ ├── components/ │ │ ├── app.module.ts │ │ ├── app.component.ts ├── angular.json ├── package.json
Angular Code Example:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Count: {{ count }}</h1> <button (click)="increaseCount()">Increase</button> `, }) export class AppComponent { count = 0; increaseCount() { this.count++; } }
Ecosystems: The Real Game-Changer
React’s Ecosystem
React’s ecosystem is enormous and diverse. Tools like Next.js make server-side rendering a breeze, while React Native lets you build mobile apps with the same codebase. However, React doesn’t dictate which tools you use, so you’ll need to assemble your stack—from routing (React Router) to state management (Redux, MobX, etc.).
Angular’s Ecosystem
Angular comes as an all-in-one package. The Angular CLI automates most tasks, and RxJS brings powerful reactive programming capabilities. Angular Universal supports server-side rendering, and you don’t need to rely on third-party tools as much as you do with React.
React vs Angular: Head-to-Head Comparison
Feature | React | Angular |
---|---|---|
Learning Curve | Moderate (flexible, but needs libraries) | Steep (opinionated and complex) |
Performance | Lightweight and fast with tools like Next.js | Heavy but stable for large apps |
Use Case | Startups, SPAs, mobile apps | Enterprise, government, complex apps |
TypeScript | Optional (can be added) | Built-in |
Community | Largest in the frontend world | Smaller but enterprise-focused |
Flexibility | High (choose your tools) | Low (all-in-one solution) |
Which Should You Choose in 2025?
Pick React if:
- You need flexibility and lightweight tools.
- Your project involves mobile apps (React Native).
- You’re building something dynamic and UI-heavy.
- You want to experiment with cutting-edge frameworks like Remix or Next.js.
Pick Angular if:
- You’re working on a large, enterprise-grade application.
- Your team prefers an all-in-one, opinionated framework.
- You want strong TypeScript integration right from the start.
- You’re building an app for government or corporate use cases.
Final Thoughts
Choosing between React and Angular depends on your project’s needs and your comfort level. React’s flexibility makes it ideal for dynamic and lightweight apps, while Angular’s structure suits large, scalable applications. Both are powerful tools—it’s all about finding the one that aligns with your goals.
In 2025, both React and Angular will remain strong contenders. React will likely dominate startups and mid-sized projects, while Angular will continue to shine in enterprise settings. Whether you’re a solo developer or part of a large team, understanding their strengths will help you make the right choice.
So, what’s your pick? Let us know in the comments below and stay tuned to CodingNaija for more coding insights!