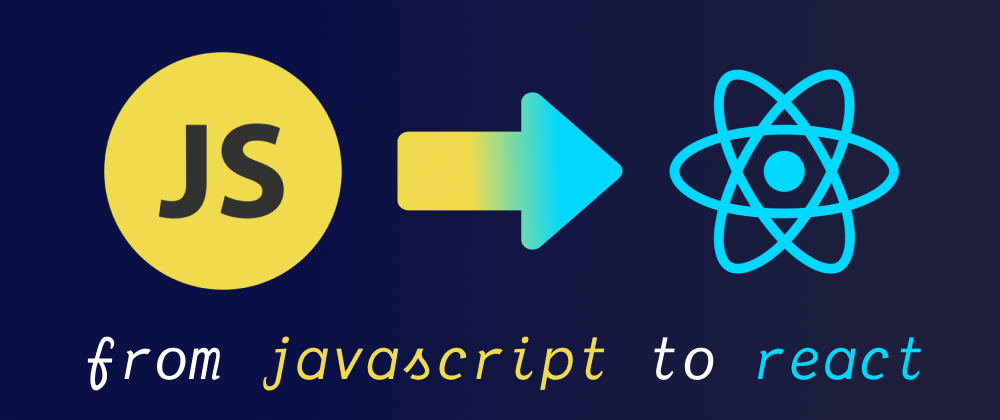
If you’re diving into the world of React, congratulations! You’re about to explore one of the most popular and powerful JavaScript libraries for building user interfaces. But before you hit the ground running, there’s something you need to know: mastering React starts with mastering JavaScript. React is built on JavaScript, and understanding its core concepts is non-negotiable if you want to succeed.
In this post, we’ll explore 10 essential JavaScript concepts that will make your React learning journey smoother and more rewarding. Let’s dive in!
1. Variables and Scoping
Why It Matters
In programming, managing data correctly depends on understanding how variables behave in different scopes. Scoping ensures variables are used within the correct context and don’t conflict with others in your code.
What to Focus On
- Use
const
for values that don’t change andlet
for those that do. Avoidvar
as it’s prone to hoisting issues. - Learn about block scope vs. function scope.
Example in JavaScript
function counterExample() { let count = 0; // Local variable function increment() { count++; console.log(count); // Logs: 1, 2, 3... } increment(); increment(); } counterExample();
2. Arrow Functions
Why It Matters
Arrow functions simplify code by offering a more concise syntax and handling the this
keyword intuitively. This makes them ideal for callbacks and functional programming.
What to Focus On
- Understand implicit returns for cleaner code.
- Learn how arrow functions inherit
this
from their surrounding context.
Example in JavaScript
const add = (a, b) => a + b; console.log(add(3, 4)); // Outputs: 7
3. Destructuring Assignment
Why It Matters
Destructuring simplifies the process of extracting values from objects and arrays, making your code cleaner and more readable.
What to Focus On
- Practice destructuring objects and arrays.
- Understand how to handle nested structures.
Example in JavaScript
const user = { name: "John", age: 30 }; const { name, age } = user; console.log(`${name} is ${age} years old.`); // Outputs: John is 30 years old.
4. The Spread and Rest Operators
Why It Matters
These operators make it easy to work with arrays and objects, especially when copying or merging data.
What to Focus On
- Use spread (
...
) to copy or combine objects and arrays. - Use rest (
...
) to gather remaining elements or properties.
Example in JavaScript
const numbers = [1, 2, 3]; const newNumbers = [...numbers, 4, 5]; console.log(newNumbers); // Outputs: [1, 2, 3, 4, 5] function sum(...args) { return args.reduce((acc, curr) => acc + curr, 0); } console.log(sum(1, 2, 3)); // Outputs: 6
5. The map()
Function
Why It Matters
The map()
method transforms arrays, making it a key tool for functional programming.
What to Focus On
- Learn how to use
map()
to process array elements. - Combine
map()
with other methods for powerful data transformations.
Example in JavaScript
const numbers = [1, 2, 3]; const squared = numbers.map(num => num * num); console.log(squared); // Outputs: [1, 4, 9]
6. Template Literals
Why It Matters
Template literals make it easy to create dynamic strings and work well for multi-line text.
What to Focus On
- Use backticks (`) for multi-line strings and variable interpolation.
- Combine template literals with expressions for flexibility.
Example in JavaScript
const name = "John"; const greeting = `Hello, ${name}!`; console.log(greeting); // Outputs: Hello, John!
7. Modules and Import/Export
Why It Matters
JavaScript modules allow you to organize code into reusable pieces, which is essential for larger projects.
What to Focus On
- Practice
export default
and named exports. - Learn the syntax for importing multiple or single modules.
Example in JavaScript
// utils.js export function greet(name) { return `Hello, ${name}`; } // main.js import { greet } from './utils.js'; console.log(greet("John")); // Outputs: Hello, John
8. Promises and Async/Await
Why It Matters
Asynchronous programming is crucial for tasks like API calls. Promises and async/await
make handling such tasks cleaner and easier.
What to Focus On
- Learn the difference between
then()
andasync/await
. - Handle errors with
try/catch
blocks.
Example in JavaScript
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error fetching data:', error); } } fetchData();
9. Event Handling
Why It Matters
Understanding how to handle events is vital for interactive applications. It ensures you can respond to user interactions effectively.
What to Focus On
- Learn common event types like
click
andchange
. - Understand how to use event listeners.
Example in JavaScript
const button = document.querySelector('button'); button.addEventListener('click', () => { alert('Button clicked!'); });
10. The this
Keyword
Why It Matters
Understanding how this
works helps you write better object-oriented code and avoid common pitfalls.
What to Focus On
- Learn how
this
behaves in different contexts. - Practice using
bind()
,call()
, andapply()
.
Example in JavaScript
const person = { name: "John", greet() { console.log(`Hello, my name is ${this.name}.`); } }; person.greet(); // Outputs: Hello, my name is John.
Final Thoughts
Starting with React is exciting, but don’t skip the fundamentals. Investing time in mastering these 10 JavaScript concepts will make React feel intuitive and empower you to build better, more efficient applications.
Take it one step at a time, and before you know it, you’ll be a React pro. Happy coding, and welcome to the React ecosystem!