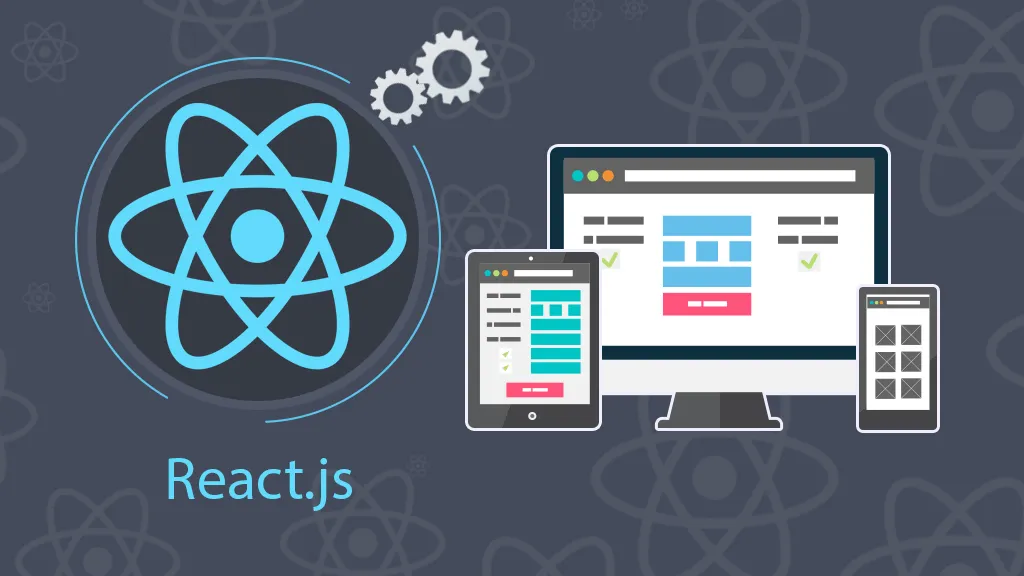
Welcome to CodingNaija, where we simplify complex coding concepts for our Naija techies and beyond. Today, we’re diving into React—a library that has revolutionized web development. If you’ve ever wanted to create sleek, dynamic websites that don’t just look good but also feel lightning-fast, React is your ticket. Let’s break it all down step by step and help you kickstart your React journey.
What is React?
React is a JavaScript library for building user interfaces. Think of it as a tool that helps you break down your website into smaller, reusable pieces called components. Created by Facebook, React focuses on making web development simple, efficient, and dynamic.
Imagine you’re building a house. Instead of constructing it brick by brick, you’re given prebuilt rooms (components) that you can assemble as you like. This modular approach is what makes React so powerful.
Here’s why React stands out:
- Declarative: You tell React what you want, and it figures out how to do it.
- Component-Based: Your app is built with small, self-contained units that you can reuse.
- Fast Updates: Thanks to the Virtual DOM, React updates only the parts of the UI that need changes, making it blazing fast.
Why Should You Learn React?
React is everywhere. From tech giants like Netflix, Airbnb, and Instagram to smaller startups, developers rely on React to deliver interactive and efficient web experiences. Learning React is like getting a VIP pass to a world of opportunities in web development.
Key Concepts to Understand
Before you dive into coding, let’s cover some foundational concepts that every React developer should know:
1. Components
Components are the building blocks of React applications. They can be thought of as reusable, self-contained pieces of UI.
- Functional Components:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
Class Components: (Older way, but still relevant in legacy code)
class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
2. JSX
JSX stands for JavaScript XML. It lets you write HTML-like syntax directly inside your JavaScript code. For example:
const element = <h1>Hello, world!</h1>;
JSX isn’t HTML, but it makes writing React components more intuitive.
3. Props and State
- Props: Short for “properties,” these are immutable data passed from a parent to a child component.
function Greeting(props) { return <p>Welcome, {props.name}!</p>; }
State: This is mutable data managed inside a component.
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }
4. Hooks
Hooks like useState
and useEffect
allow you to manage state and lifecycle in functional components. They’re the modern way to write React code, so you’ll use them a lot.
5. Virtual DOM
The Virtual DOM is a lightweight copy of the real DOM. React uses it to figure out the minimal changes needed to update your UI, making updates super efficient.
Setting Up Your React Environment
Let’s get you started with your first React project.
Option 1: Use Create React App (The Easy Way)
This is the quickest way to get up and running:
- Install Node.js: Download and install Node.js from nodejs.org.
- Create a New React App:
npx create-react-app my-app cd my-app npm start
- Open http://localhost:3000 in your browser, and you’ll see your first React app live!
Option 2: Manual Setup (For Curious Minds)
- Initialize a Project:
mkdir react-manual && cd react-manual npm init -y
- Install Dependencies:
npm install react react-dom npm install --save-dev webpack webpack-cli babel-loader @babel/core @babel/preset-env @babel/preset-react
- Configure Webpack: Create a
webpack.config.js
file:
module.exports = { entry: './src/index.js', output: { path: __dirname + '/dist', filename: 'bundle.js' }, module: { rules: [{ test: /\.js$/, use: 'babel-loader' }] }, };
- Write Code: Create
src/index.js
:import React from 'react';
import ReactDOM from 'react-dom'; const App = () => <h1>Hello, React!</h1>; ReactDOM.render(<App />, document.getElementById('root'));
- Run Your App: Use Webpack to build and serve your app.
Your First React Project: Build a Counter
Let’s put your knowledge to the test with a simple counter app.
Features:
- Increment and decrement the counter.
- Reset the counter.
- Change text color based on the counter value (e.g., red for negative).
Here’s the code:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <h1 style={{ color: count < 0 ? 'red' : 'green' }}>Count: {count}</h1> <button onClick={() => setCount(count + 1)}>Increment</button> <button onClick={() => setCount(count - 1)}>Decrement</button> <button onClick={() => setCount(0)}>Reset</button> </div> ); } export default Counter;
Save this component, render it in your App.js
, and see your counter in action!
Next Steps
React is a vast ecosystem. Here’s what to explore next:
- Routing: Learn how to navigate between pages with React Router.
- State Management: Dive into Redux or Context API for managing global state.
- Styling: Experiment with CSS frameworks like TailwindCSS or styled-components.
- Advanced Features: Get familiar with Hooks like
useReducer
anduseMemo
.
Conclusion
React is more than just a library; it’s a gateway to building modern web applications. By understanding its core concepts and practicing regularly, you’ll unlock a world of possibilities. Remember, coding is a journey, and every line of code brings you closer to mastery.
Let us know how your first React project went in the comments below. Happy coding!