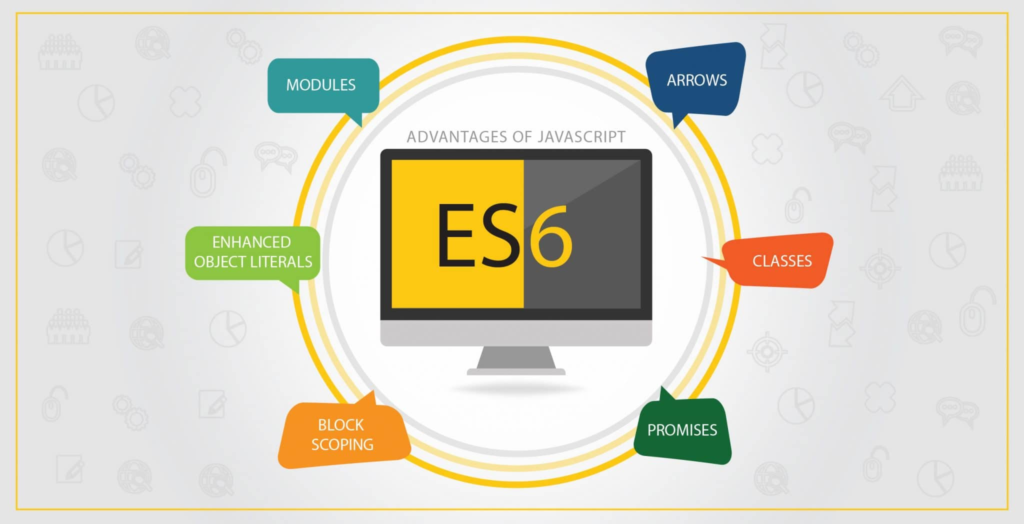
JavaScript is one of the most widely used programming languages today, powering everything from dynamic web applications to server-side logic. But there was a time when JavaScript had its fair share of struggles. Then came ES6 (ECMAScript 2015), a landmark update that completely changed the game for developers. If you’ve ever wondered why ES6 gets so much hype, buckle up—this post will walk you through the features that made developers fall in love with JavaScript all over again.
1. let
and const
: Block-Scoped Variable Declarations
Before ES6, we only had var
to declare variables, which came with its own set of headaches. Variables declared with var
had function scope, not block scope, leading to unexpected bugs.
ES6 introduced let
and const
, which are block-scoped. This means they’re confined to the block where they’re defined. let
is used for variables that can change, while const
is for constants that can’t be reassigned.
Example:
if (true) { let age = 25; const name = "CodingNaija"; } // console.log(age); // Error: age is not defined // console.log(name); // Error: name is not defined
Why it matters: Fewer bugs caused by accidental variable overwrites and scope leakage.
2. Arrow Functions: Cleaner Syntax
Writing functions in JavaScript used to be verbose and confusing when it came to this
binding. Arrow functions came to the rescue with a concise syntax and automatic this
binding.
Example:
// Traditional function const double = function (num) { return num * 2; }; // Arrow function const doubleArrow = (num) => num * 2; console.log(doubleArrow(4)); // Output: 8
Why it matters: Less boilerplate code and easier handling of this
.
3. Template Literals: Dynamic Strings Made Easy
Before ES6, string concatenation in JavaScript was clunky and error-prone. Enter template literals, which allow embedded expressions and multi-line strings.
Example:
const name = "Naija Dev"; const greeting = `Hello, ${name}! Welcome to CodingNaija.`; console.log(greeting); // Multi-line strings const poem = `Roses are red, Violets are blue, JavaScript rocks, And so do you!`; console.log(poem);
Why it matters: Simplifies string operations, making code cleaner and more readable.
4. Destructuring: Simplified Data Extraction
Extracting values from objects and arrays used to be a repetitive task. Destructuring in ES6 allows you to unpack these values into variables in a single line.
Example:
// Array destructuring const coordinates = [10, 20]; const [x, y] = coordinates; // Object destructuring const user = { name: "Alice", age: 30 }; const { name, age } = user; console.log(name, age); // Output: Alice 30
Why it matters: Cleaner and faster handling of structured data.
5. Modules: A Native Way to Organize Code
Before ES6, developers relied on external libraries like RequireJS or made do with messy global variables for modularization. ES6 introduced a native module system using import
and export
.
Example:
// module.js export const greet = name => `Hello, ${name}`; // main.js import { greet } from "./module.js"; console.log(greet("Naija Dev")); // Output: Hello, Naija Dev
Why it matters: Simplifies dependency management and encourages cleaner, modular code.
6. Promises: A Cleaner Way to Handle Asynchronous Code
Asynchronous JavaScript was a mess before ES6, with deeply nested callbacks often referred to as “callback hell.” Promises provide a cleaner, more structured way to handle async tasks.
Example:
// Fetching data with Promises fetch("https://api.example.com/data") .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error("Error fetching data:", error));
Why it matters: Improved readability and error handling for asynchronous operations.
7. Classes: Object-Oriented JavaScript Made Simple
Object-oriented programming in JavaScript before ES6 involved convoluted prototypes. ES6 introduced classes, making it easier to define objects and inheritance.
Example:
class Animal { constructor(name) { this.name = name; } speak() { console.log(`${this.name} makes a sound.`); } } const dog = new Animal("Dog"); dog.speak(); // Output: Dog makes a sound.
Why it matters: Makes JavaScript more approachable for developers from other OOP languages.
8. Spread and Rest Operators: Versatility at Its Best
The spread (...
) and rest (...
) operators simplify working with arrays and objects in JavaScript.
Example:
// Spread operator const arr1 = [1, 2]; const arr2 = [3, 4]; const combined = [...arr1, ...arr2]; console.log(combined); // Output: [1, 2, 3, 4] // Rest operator function sum(...numbers) { return numbers.reduce((total, num) => total + num, 0); } console.log(sum(1, 2, 3, 4)); // Output: 10
Why it matters: Makes handling dynamic data structures simpler and more expressive.
Why the ES6 Hype?
ES6 wasn’t just an upgrade—it was a paradigm shift. It addressed long-standing pain points, introduced modern features, and set the stage for future updates like async/await (ES8). Whether you’re new to JavaScript or an experienced dev, ES6 is the foundation of the language as we know it today.
So, if you’ve ever wondered why developers can’t stop raving about ES6, now you know: it’s because these features truly changed the game.
Ready to Level Up? If you’re inspired to dive deeper into JavaScript and ES6, CodingNaija has got your back! Share your thoughts, questions, or favorite ES6 features in the comments below.