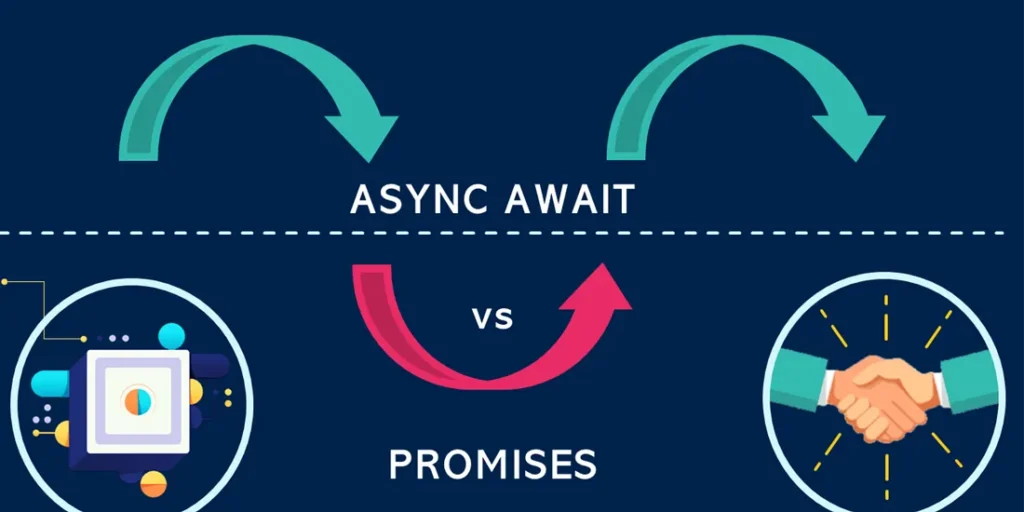
As a JavaScript developer, you’ve likely faced the question: “Should I use Promise chaining or async/await?” Both are powerful tools for handling asynchronous code in JavaScript, but each has its strengths and ideal use cases. Let’s break this down and explore the differences so you can confidently choose the right approach for your projects.
What Are We Dealing With?
Before diving into comparisons, here’s a quick recap of what Promise chaining and async/await are.
Promise Chaining
Promise chaining is a technique where you link multiple .then()
calls together to handle asynchronous tasks in sequence. Each .then()
passes its result to the next one, creating a streamlined flow of operations.
Example:
fetch("/api/user") .then((response) => response.json()) .then((user) => updateUserAccess(user)) .then((updatedUser) => console.log("Updated User:", updatedUser)) .catch((error) => console.error("Error:", error));
Async/Await
Async/await was introduced in ES2017 to simplify working with Promises. It allows you to write asynchronous code in a style that resembles synchronous code, making it easier to read and debug.
Example:
async function fetchAndUpdateUser() { try { const response = await fetch("/api/user"); const user = await response.json(); const updatedUser = await updateUserAccess(user); console.log("Updated User:", updatedUser); } catch (error) { console.error("Error:", error); } }
Why the Debate?
Both approaches achieve the same goal: handling asynchronous operations. But developers often argue over which is better for specific environments like React or Node.js. Here’s the deal:
React Loves Async/Await
React encourages clean, declarative, and readable code. Async/await fits perfectly into this philosophy because:
- Readability: Code written with async/await looks more like synchronous code, which is easier to follow.
- Error Handling: Using
try...catch
makes it simple to handle errors in a structured way, which is crucial for updating UI components based on state changes.
For example, fetching data in a React useEffect
hook often involves async/await:
useEffect(() => { const fetchData = async () => { try { const response = await fetch("/api/data"); const data = await response.json(); setData(data); } catch (error) { console.error("Error fetching data:", error); } }; fetchData(); }, []);
Node.js’s Affinity for Promise Chaining
Node.js applications often deal with tasks like API calls, database queries, and file I/O. Promise chaining integrates seamlessly into these workflows because:
- Functional Style: Middleware systems in Node.js, like Express, align naturally with chained operations.
- Composability: Promise chaining makes it easy to modularize and reuse individual
.then()
blocks.
For instance, here’s how you might handle multiple database operations:
getUserById(userId) .then((user) => fetchUserOrders(user)) .then((orders) => processOrders(orders)) .catch((error) => console.error("Error:", error));
Key Differences
Here’s a side-by-side comparison to help you understand the nuances:
Factor | Async/Await | Promise Chaining |
---|---|---|
Readability | Cleaner and easier to read for sequential tasks | Slightly harder to read with nested .then() |
Error Handling | Simple with try...catch | Relies on .catch() for error propagation |
Concurrency | Handles sequential tasks well | Can handle concurrent operations with Promise.all |
Best Fit | UI-focused workflows (e.g., React) | Backend tasks and pipelines (e.g., Node.js) |
The Perception: Is Async/Await for React and Promise Chaining for Node.js?
There’s a common belief that async/await is better for React, while Promise chaining shines in Node.js. This perception isn’t entirely wrong, but it’s more of a guideline than a hard rule.
Why This Perception Exists
- React:
- React’s declarative nature pairs well with async/await, especially for handling state updates and side effects like data fetching.
- Complex error handling is simpler with
try...catch
, which is common in React components.
- Node.js:
- Promise chaining fits well into the functional programming patterns often seen in Node.js applications.
- Middleware workflows and concurrent tasks, like database queries, are easier to manage with chaining.
Reality Check
- Both methods work in any environment.
- Async/await is now widely used in modern Node.js projects.
- You can use Promise chaining in React for cases like fetching data in batches:
Promise.all([ fetch("/api/user").then((res) => res.json()), fetch("/api/posts").then((res) => res.json()) ]) .then(([user, posts]) => { setUser(user); setPosts(posts); }) .catch((error) => console.error("Error:", error));
Final Thoughts
Both async/await and Promise chaining are essential tools in a JavaScript developer’s arsenal. The choice between them depends on context, readability, and the task at hand:
- Use async/await for clean, sequential code and simpler error handling.
- Use Promise chaining for modular, pipeline-like workflows and concurrent operations.
At the end of the day, the best tool is the one that makes your code clearer and your job easier. Choose wisely, Naija coders!
Got questions or thoughts on this? Drop them in the comments below—let’s keep the coding conversation going! Happy coding!